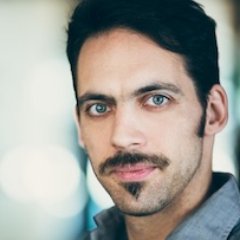
Articles
A collection of my articles on web development, Ruby on Rails, and more.
Form Objects, the missing abstraction in Rails
Stop putting the right code in the wrong place!
It’s easy to get carried away using Rails scaffolding, but sometimes a Plain Old Ruby Object (PORO) is more than appropriate. And Form Objects are very good at keeping models lean, controllers sharp, and grouping related code together in one object that’s easy to test.
Why Testing Improves Coding
Last Thursday, I attended “Good enough testing”, an online workshop by Lucian Ghinda, curator of the Short Ruby Newsletter. I’m glad that I saw the registration soon after it was posted, because the workshop was sold out in less than a day!
I took some time to combine the knowledge from the workshop with what I’ve learned over the years, in blogs and books.
Rails validation contexts
Rails validators are expressive and powerful, yet easy to use and extend.
One interesting feature is that validators can run only when a condition is met (using if:
and unless:
), or in selected contexts, using on:
.
But did you know that you can also define custom contexts? Here’s how to do it, and why that might be useful.
Silencing bundler in an inline script
Bundler can be used in inline scripts, which is awesome! Here is how to silence Bundler so that your script doesn’t start with Bundler’s output, and a workaround for a little gotcha when silencing output.
Rspec tips and tricks
Running tests is crucial but can be time-consuming, lenghtening the feedback loop between code and side-effects. Here are some ways to run only the tests you need using Rspec, to stop wasting time staring at the screen.
Run commands using a different ruby version using rbenv
While building a script to test ruby version upgrades in a rbenv
-enabled system, I noticed that running bundle install
from within a ruby script uses the script’s version of ruby instead of the one defined in the directory.
ActiveStorage fallback for disk storage
I’m working on an app using DiskService
for ActiveRecord
storage. To debug a gnarly issue, I had to copy the production database to development, but didn’t want to download the blobs themselves. Here’s how to serve a fallback image when an ActiveStorage
file is missing from the storage
folder.
How to setup Fontawesome in a Bridgetown website
The Bridgetown documentation is well-written but doesn’t explain everything needed to add FontAwesome, so I documented the steps while converting my website.
Reusable filtering concern for Rails models
I wanted to implement a generic filtering concern for Rails models, and decided to do things a bit differently from what Justin Weiss and Fabio Pitino did.
Storing settings as JSONB using Rails and PostgreSQL
You can store native JSON data in Postgres databases, and Rails now allows taking advantage of that format.
Pretty URLs with Rails 6, FriendlyId, and CanCanCan
While building a Rails 6 application, I needed to generate pretty URLs using a model name. I also wanted these URLs to change when the model name was changed, and redirect to the latest URL if someone visited the old URL. As an added constraint, I’m using the CanCanCan authorization gem to handle rights management. Here is the setup I came up with to redirect old URLs while preserving most of the gem’s expected behaviour.